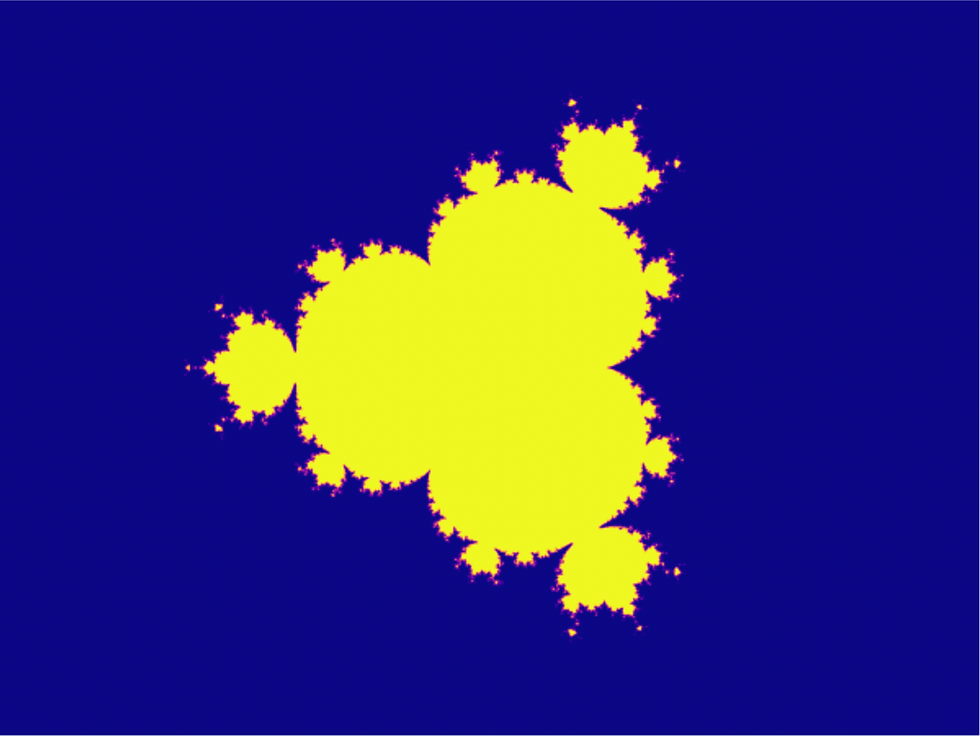
Here, you can see the visualization of a Mandelbrot fractal with exponent 4.
import matplotlib.pyplot as plt
import numpy as np
import math
np.warnings.filterwarnings("ignore")
c = complex_matrix(-2, 2, -1.5, 1.5, pixel_density=512)
def complex_matrix(xmin, xmax, ymin, ymax, pixel_density):
re = np.linspace(xmin, xmax, int((xmax - xmin) * pixel_density))
im = np.linspace(ymin, ymax, int((ymax - ymin) * pixel_density))
return re[np.newaxis, :] + im[:, np.newaxis] * 1j
def is_stable(c, e, num_iterations):
z = 0
for _ in range(num_iterations):
z = z ** e + c
return abs(z) <= 2
def visualize(exponent = 2, num_iterations=20) :
plt.imshow(is_stable(c, exponent, num_iterations), cmap='plasma' )
plt.gca().set_aspect("equal")
plt.axis("off")
plt.tight_layout()
plt.show()
visualize(exponent=4)
Comments